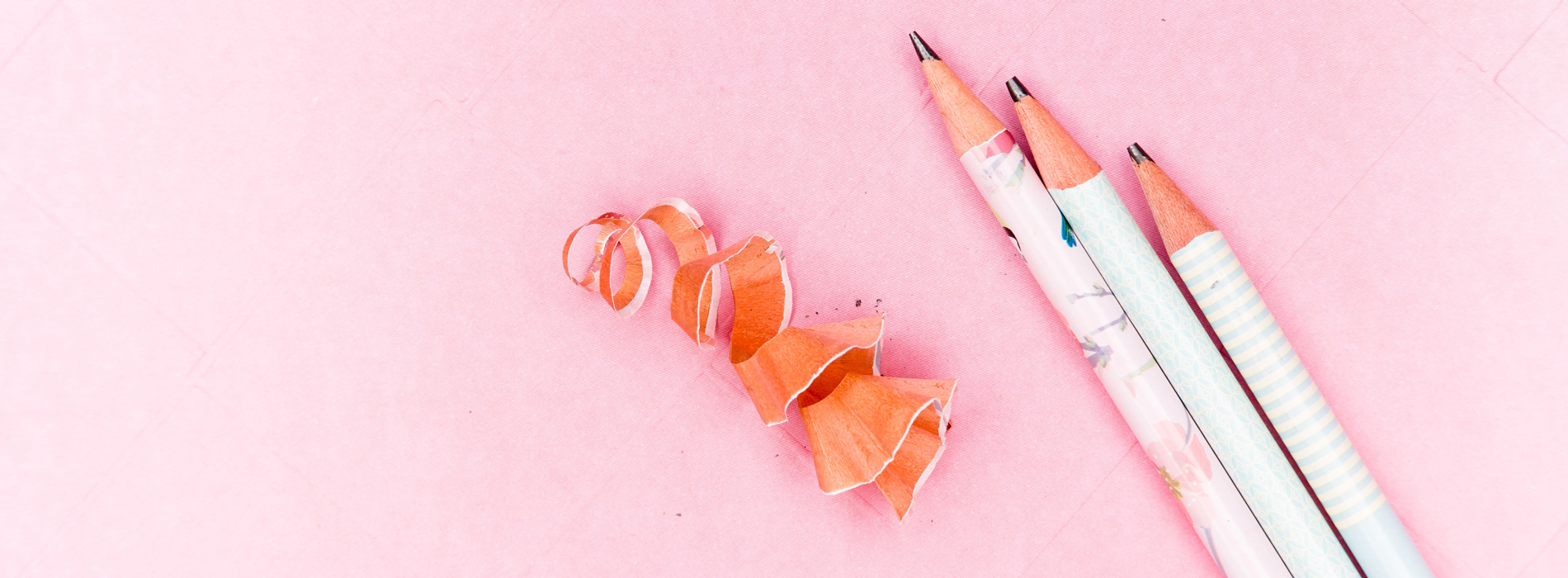
Xaml design time data: Radical.Design live experience
Power to the UI designers
Now we have one requirement, we need to give the ability to the UI designers to input values at design time letting them change values dynamically directly in the designer without any need to recompile anything, to understand Xaml markup or, even worse, to understand C# code.
First of all let’s complete the sample we begun the last time:
- edit the DesignTimePersonViewModel to expose also the LastName property adding the following code snippet to the class constructor:
this.Expose( vm => vm.LastName ) .WithStaticValue( "Servienti" );
- Add a new TextBox bound to the newly exposed property to the MainWindow;

We want to give the ability to edit directly in the designer both the FirstName property and the LastName one, let’s go back to the DesignTimePersonViewModel:
- Add 2 public properties to the class:
public String DesignTime_LastName { get { return this.GetPropertyValue( () => this.DesignTime_LastName ); } set { this.SetPropertyValue( () => this.DesignTime_LastName, value ); } } public String DesignTime_FirstName { get { return this.GetPropertyValue( () => this.DesignTime_FirstName ); } set { this.SetPropertyValue( () => this.DesignTime_FirstName, value ); } }
These are standard CLR properties that has built-in support for INotifyPropertyChanged, and lots of other stuff that are out of the scope of this post.
-
Change the public constructor like this:
public DesignTimePersonViewModel() { this.Expose( vm => vm.FirstName ) .WithLiveValue( () => this.DesignTime_FirstName ); this.Expose( vm => vm.LastName ) .WithLiveValue( () => this.DesignTime_LastName ); }
Go back to the designer, select the Grid using the mouse and select the Grid DataContext using the breadcrumb at the bottom of the designer:
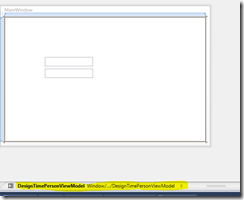
The textboxes are empty but take a look a the properties editor:
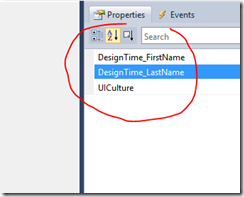
As you can see there are the 2 properties we have defined on the DesignTimePersonViewModel class, edit one of the property directly in the property editor :-)

We have live properties, cool. But we can do much more, this is just the tip of the iceberg.
Stay tuned!
.m