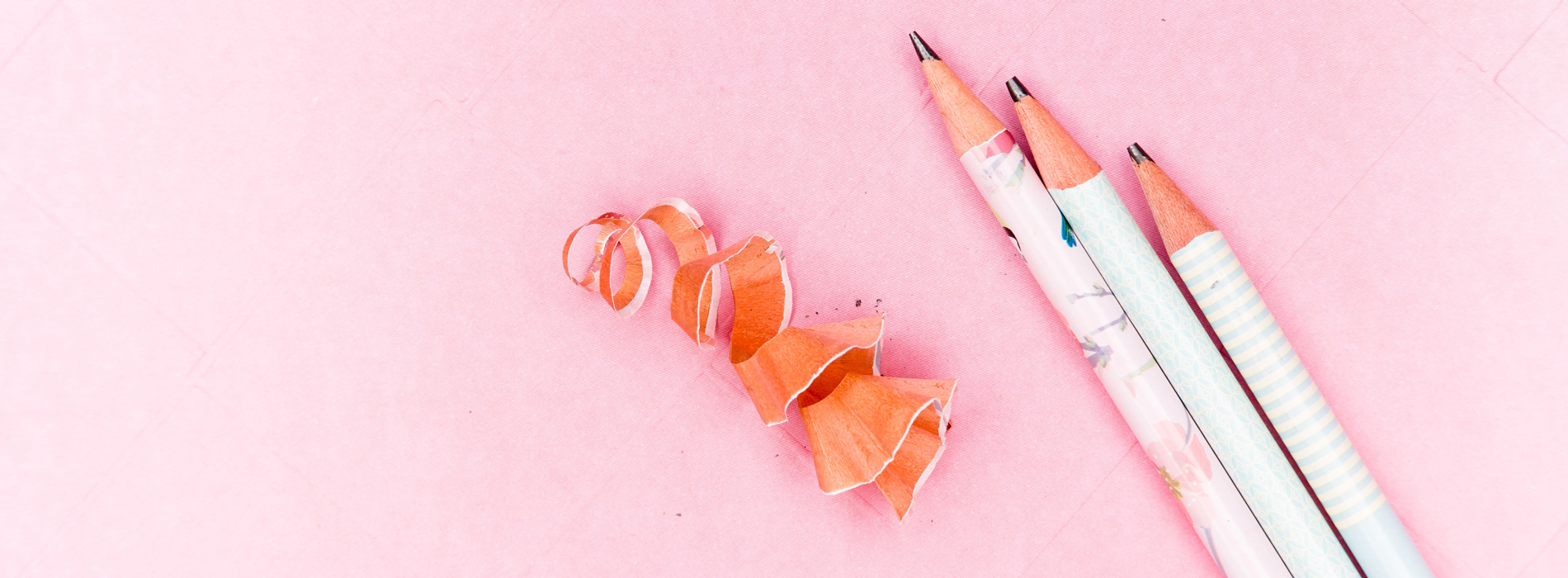
Jason v0.2.1.0: retry policies
As promised here we are :-)
One of our customer, using Jason, has a requirement to implement the concept of retry during the standard command execution flow. basically they have a situation where some infrastructure/transient exceptions can be raised during the command execution request and they want to be able to retry without bothering the client if not needed.
Retry Policy
In Jason v0.2.1.0, at the time of this writing in beta, we introduced the concept of retry policy; a retry policy is something that implements the ICommandExecutionRetryPolicy interface:
public interface ICommandExecutionRetryPolicy { object Execute( Object command, Func<ICommandHandler> handlerFactory ); }
And can be injected into the Jason configuration in the following way:
jasonConfig.UsingAsRetryPolicy<MyCustomPolicy>();
Where MyCustomPolicy is a class that implements ICommandExecutionRetryPolicy. In the above sample the policy is registered in the container at initialization time and used by the various Jason entry point to handle all the retry logic.
There is one built-in retry policy in Jason that can be used to handle retries in case of failure:
jasonConfig.UsingAsRetryPolicy( new DelegateCommandExecutionRetryPolicy() { DefaultRetryDelay = 200, ShouldRetry = e => e.Retry = ( e.Error is NotSupportedException && e.CurrentRetryCount < 5 ) } );
In this case the policy is still registered in the container using the supplied instance, at runtime if a failure occurs the policy is queried to determine if a retry should be attempted.
.m