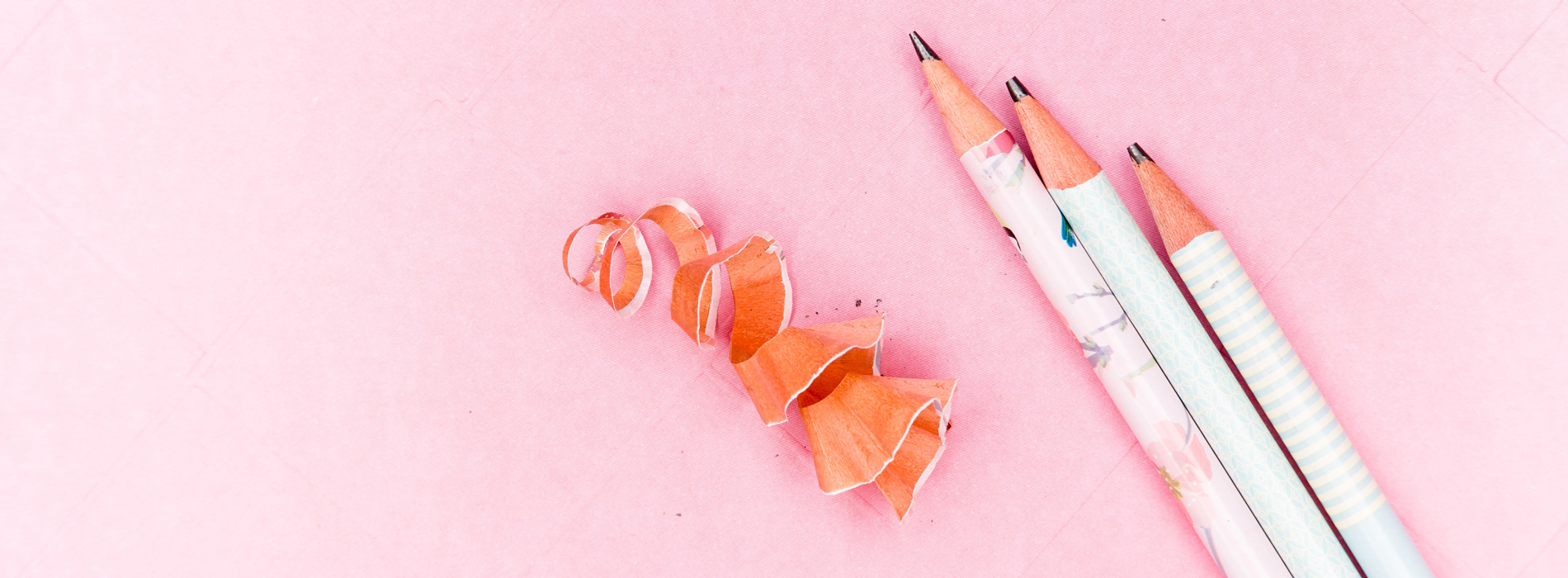
Jason: MVC WebAPI Hosting
Last time we have seen what Jason is, the big picture, but we still miss a lot of details and as you may have noticed even the samples of the introduction post miss some important details.
The important things we discovered can be summarized as follows:
- we have understood the commanding pattern exposed by Jason;
- we have understood how Jason behaves from the client perspective;
- we have understood that we can host Jason in a WebAPI controller or using a WCF service;
Jason setup in MVC WebAPI
Let us start from the newest stuff but much simpler WebAPI implementation, first of all create a new MVC WebAPI project and grab a reference to the Jason.WebAPI package from nuget.
What do you get in the package?
- A built-in JasonController WebAPI controller;
- The default configuration implementation;
- The default command handler provider;
- 1 built-in command (EchoCommand) for testing purpose and its related command handler;
2, 3, go… (cit.)
One you have added the nuget package all we have to do is to configure Jason, I’d like to improve the configuration so if you have nay idea let me know.
Open up the Global.asax file of your project and:
- Jason relies on a Inversion of Control framework to work but has no explicit dependency on any specific IoC container, since the requirements are really basic I preferred to expose a simple API to let the user wire Jason with his favorite tool:
var windsor = new WindsorContainer();
windsor.Register( Component.For<IServiceProvider>().Instance( new ServiceProviderWrapper( windsor ) ) );
windsor.Register( Component.For<ICommandHandlersProvider>().ImplementedBy<DefaultCommandHandlersProvider>() );
windsor.Register( Component.For<JasonController>().LifeStyle.Is( LifestyleType.Transient ) );
Right after the MVC initialization code we are creating a new instance of Castle Windsor adding a bunch of components.
- After setting up the IoC container we can setup the Jason configuration:
var jasonConfig = new JasonWebAPIConfiguration
(
pathToScanForAssemblies: Path.Combine( AppDomain.CurrentDomain.BaseDirectory, "bin" ),
jsonTypeNameHandling: TypeNameHandling.Objects
)
{
RegisterAsTransient = ( c, i ) =>
{
windsor.Register
(
Component.For( c )
.ImplementedBy( i )
.LifeStyle.Is( Castle.Core.LifestyleType.Transient )
);
}
};
jasonConfig.Initialize();
The configuration needs 3 different things:
- a path that the internal type scan engine utilizes to find all the commands and all the handlers;
- A type name handling behavior to handle json commands deserialization (only used if incoming content-type is application/json);
- A Func<Object, Object> used to ask to the external IoC container to register something in a transient manner;
The call to Initialize wires up everything and prepares Jason for the first run.
- The last thing to do is to instruct the WebAPI framework to use our IoC implementation to resolve dependencies:
GlobalConfiguration.Configuration.DependencyResolver = new DelegateDependencyResolver()
{
OnGetService = t =>
{
if ( windsor.Kernel.HasComponent( t ) )
{
return windsor.Resolve( t );
}
return null;
},
OnGetServices = t =>
{
if ( windsor.Kernel.HasComponent( t ) )
{
return windsor.ResolveAll( t ).OfType<Object>();
}
return new List<Object>();
}
};
here I am using a really trivial IDependencyResolver implementation to delegate to the user code the dependency resolve process and let the resolver only handle the wiring between the WebAPI infrastructure and our favorite IoC container.
Now that everything is set up we can test our brand new Jason infrastructure:
- Startup the newly created WebAPI application pressing F5 in Visual Studio;
- fire up a new instance of Fiddler and go to the “composer”:
Add a call to Jason controller and remember to set the post content type, in our sample json but also xml is fully supported.
Finally add a boy to the posted http request:
Execute
As soon as you press the execute button the Jason infrastructure is invoked from the WebAPI application and you get the expected response:
Server side what is happening is that the specialized command handler for the EchoCommand is invoked by the Jason infrastructure, and has the opportunity to do some work and provide a response:
public class EchoCommandHandler : AbstractCommandHandler<EchoCommand>
{
protected override object OnExecute( EchoCommand command )
{
return new EchoCommandResponse()
{
Message = String.Format( "Echo: {0}", command.Message )
};
}
}
The cool thing is that the whole wiring is done by the infrastructure, thus we can:
- Create a bunch of commands in your assemblies;
- Create the dedicated command handler for each command;
- drop all the stuff in the bin folder of the WebAPI application and restart the application;
We can now use the newly created commands directly from our really cool JavaScript application using WebAPI as a backhand to host command handlers.
.m