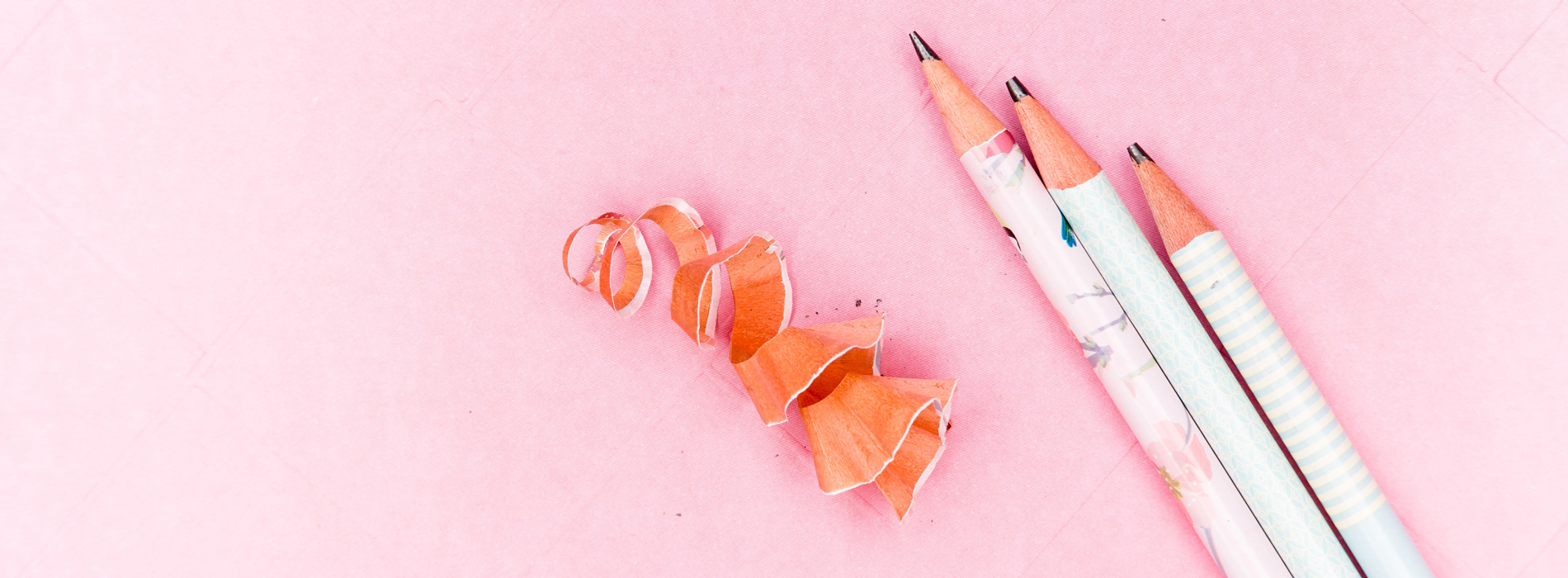
Radical.Windows.Presentation: runtime conventions
We have already introduced some of the runtime conventions used by Radical.Windows.Presentation. Radical.Windows.Presentation has also a lot of runtime conventions mainly related to two different areas:
- View – ViewModel relation;
- UI Composition;
Here they are:
public interface IConventionsHandler { Func<Type, Type> ResolveViewModelType { get; set; } Func<Type, Type> ResolveViewType { get; set; } Func<Object, Window> FindHostingWindowOf { get; set; } Predicate<DependencyObject> ViewHasDataContext { get; set; } Action<DependencyObject, Object> SetViewDataContext { get; set; } Func<DependencyObject, Object> GetViewDataContext { get; set; } Action<DependencyObject, Object> AttachViewToViewModel { get; set; } Func<Object, DependencyObject> GetViewOfViewModel { get; set; } Func<DependencyObject, Action<DependencyObject>, DependencyObject> TryHookClosedEventOfHostOf { get; set; } Func<FrameworkElement, Boolean> IsHostingView { get; set; } Action<DependencyObject> AttachViewBehaviors { get; set; } }
ResolveViewModelType
The first convention is used internally by the ViewResolver and given the view type returns the ViewModel type for the given view, the default behavior is that the view model is in the same namespace of the view and has the same type name suffixed with “Model” (e.g.: MainView and MainViewModel)
ResolveViewType
The ResolveViewType convention is currently under development and not used, but basically does the opposite stuff, using the same default behavior, as the ResolveViewModelType convention.
FindHostingWindowOf
This convention is currently used to find the Window that hosts a given view model, quite certainly this convention will be changed in the near future to support the fact that in Windows 8 Metro style apps a Window object does not exists.
This task is performed finding the current view of the given view model (using another convention) and then reverse walking the visual tree looking fir the first Window.
The convention accomplish two needs:
- A view model can implement the IExpectViewClosingCallback and the IExpectViewClosedCallback in order to intercept the fact that the hosting Window is closing or has been closed and since we support UI Composition features a view model can be a view model attached to a UserControl that is runtime “inserted” into the visual tree of an existing Window;
- The UI Composition region service, in order to satisfy the above requirement, each time setups a new region need to find the hosting window in order to attach the closing and closed events;
ViewHasDataContext, SetViewDataContext and GetViewDataContext
The ViewHasDataContext convention simply checks if the given view DataContext property is not null, this convention accepts a DependencyObject because in WPF the DataContext property is not defined on a single root object but is defined on FrameworkElement and on FrameworkContentElement.
SetViewDataContext and GetViewDataContext respectively sets and gets the DataContext of the given view.
AttachViewToViewModel and GetViewOfViewModel
Internally the Radical.Windows.Presentation MVVM and UI Composition toolkit needs to know runtime View – ViewModel relations in order to know that given a ViewModel instance the corresponding View instance is certainly a specific instance.
To achieve that the ViewResolver once has resolved both the required instances calls the AttachViewToViewModel convention in order to store the view reference in the view model instance (the view model is already stored in view instance using the DataContext property).
By design this works out-of-the-box because the AbstractViewModel type implements the IViewModel interface that has a View property internally used for this tasks. If the user does not like this behavior or cannot inherit from the AbstractViewModel type, nor implement the IViewModel interface, can replace this convention in order to store somewhere else the required relation.
The same logic is used by the GetViewOfViewModel convention that is required to retrieve the stored relation.
TryHookClosedEventOfHostOf
This is really internal and is used by the region service engine to attach the closed event of the hosting window, if any, in order to cleanup stuff when the window closes.
IsHostingView
The IsHostingView convention is internally used by the Region base class to determine if a given visual element can be considered a View.
AttachViewBehaviors
The AttachViewBehaviors convention can be hooked by the framework user if there is a requirement to attach behaviors (System.Windows.Interactivity.Behavior<T>) whenever a view is resolved by the ViewResolver.
Conclusion
As you can see this post is mainly a documentation post not so interesting, well at least for me, do not worry in the next post we’ll introduce the UI Composition features supported by Radical.Windows.Presentation.
Stay tuned.
.m