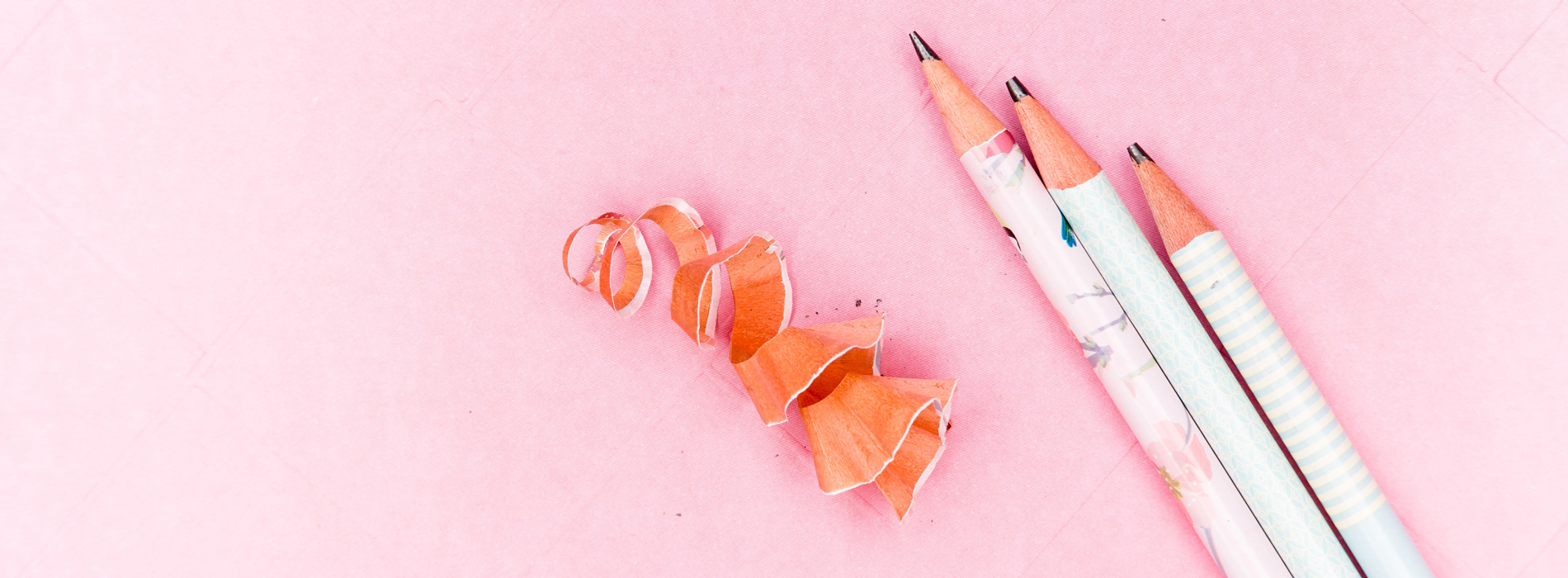
Radical 1.0.1 (Vacuum): BusyStatusManager e AsyncWorker

Una piccola lamentela che so essere condivisa da molti: *****… ma non c’è una mazza su Silverlight… :-)AsyncWorker
Anche su Silverlight e Windows Phone è possibile fare questo:
Che grazie ai behavior e agli adorner ci consente di iniettare uno “Wait Screen” per dare feedback all’utente.public class MainPageViewModel : AbstractViewModel { public MainPageViewModel() { this.SampleCommand = DelegateCommand.Create() .OnExecute( o => { AsyncWorker.Using( new { Now = DateTime.Now, Parameter = o, Dispatcher = Deployment.Current.Dispatcher } ) .Configure( cfg => { cfg.Before = e => this.IsBusy = true; cfg.After = e => this.IsBusy = false; } ) .Execute( e => { Thread.Sleep( 1000 ); e.Argument.Dispatcher.BeginInvoke( () => { this.SampleText = String.Format( "{0} - {1}", e.Argument.Parameter, e.Argument.Now.ToLongTimeString() ); } ); } ); } ); } public ICommand SampleCommand { get; private set; } private String _sampleText = null; public String SampleText { get { return this._sampleText; } set { if( value != this.SampleText ) { this._sampleText = value; this.OnPropertyChanged( () => this.SampleText ); } } } private Boolean _isBusy = false; public Boolean IsBusy { get { return this._isBusy; } private set { if( value != this.IsBusy ) { this._isBusy = value; this.OnPropertyChanged( () => this.IsBusy ); } } } }
BusyStatusManager
Uno “Wait Screen” altro non è che un Adorner totalmente gestito altrove il cui ciclo di vita è tipicamente controllato da una proprietà “IsBusy”. La cosa decisamente comoda del BusyStatusManager è che espone un semplicissimo meccanismo, basato su composition, che consente al designer di decidere quale sia il layout dello “Wait Screen”:
Il BusyStatusManager è “appiccicabile” su qualsiasi controllo e visualizzerà lo “Wait Screen” esattamente sovrapposto allo UIElement su cui è applicato:<phoneNavigation:PhoneApplicationPage xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:phoneNavigation="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone.Controls.Navigation" xmlns:behaviors="clr-namespace:Topics.Radical.Windows.Behaviors;assembly=Radical.Windows" xmlns:converters="clr-namespace:Topics.Radical.Windows.Converters;assembly=Radical.Windows" x:Class="Vacuum.Sample.MainPage" xmlns:controls="clr-namespace:Topics.Radical.Windows.Controls;assembly=Radical.Windows"> <phoneNavigation:PhoneApplicationPage.Resources> <converters:BooleanBusyStatusConverter x:Key="bbs" /> phoneNavigation:PhoneApplicationPage.Resources> <controls:AdornerLayer> <Grid x:Name="LayoutRoot" behaviors:BusyStatusManager.Status="{Binding Path=IsBusy, Converter={StaticResource bbs}}" Background="{StaticResource PhoneBackgroundBrush}"> <behaviors:BusyStatusManager.Content> <Border Background="Red"> <TextBlock Text="Please wait...:-)" HorizontalAlignment="Center" VerticalAlignment="Center" /> Border> behaviors:BusyStatusManager.Content> Grid> controls:AdornerLayer> phoneNavigation:PhoneApplicationPage>
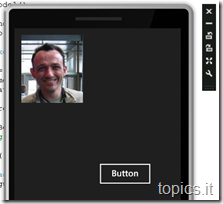
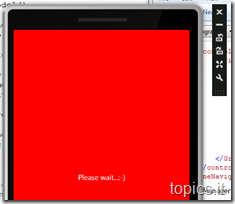
ICommandSource
Windows Phone 7 a oggi è basato su Silverlight 3 e quindi non c’è supporto da parte dei controlli per ICommandSource. Radical offre, per la sola parte Windows Phone, un behavior per aggiungere questo supporto:
Ovviamente c’è anche CommandParameter :-)<Button behaviors:ButtonBase.Command="{Binding Path=SampleCommand}" Content="My Sample Button" />
Happy SilverPhoning :-)
.m